Coupled Differential Equations Continued!
Contents
Coupled Differential Equations Continued!#
Recap for coupled differential equations:#
Coupled homogeneous 1st order ODEs:
\(\vec{y'} = \arr{A} \vec{y}\) where \(\vec{y}\) is a vector of unknown functions.
\(\vec{y} = [y_1, y_2, y_3, ... y_n]^T\) represents an \(n^{th}\) order system with \(n\times n \ \arr{A}\)
We assumed that \(\vec{x}e^{\lambda t}\) was a solution, then showed that it was.
We proved that the eigenvalues and eigenvectors of \(\arr{A}\) yield the solution
In the last class, we looked at examples of coupled \(1^\circ\) ODEs with real, distict eigenvalues.
Now let’s look at complex eigenvalues#
\(\underline{\text{Ex}}\):
Can you guess what the solutions are going to look like?
Find eigenvalues of \(\arr{A}\):
Find eigenvectors
Set \((\arr{A} - \lambda\arr{I})\vec{x}^{(1)} = \vec{0}\)
For \(\lambda_1 = 2i\)
For \(\lambda_2 = -2i\)
\(\therefore\) General solution is:
Not very useful since it’s imaginary
We will again use Euler’s Formula:
Looking at part of the solution:
Can we form real solutions from the imaginary solutions?
\(\implies\) Let’s try multiplying sum of imaginary solutions by \(\frac{1}{2}\)
\(\therefore \vec{y} = c_1 \vec{a} + c_2 \vec{b}\) is a real representation of the general solution
OR
General solution for complex eigenvalues#
We don’t have to go through all of this work every time!
For pairs of complex conjugate eigenvalues \(\lambda\pm \omega i\) with eigenvectors \(\vec{x}=\vec{a}\pm \vec{b}i\) (the eigenvectors are also complex conjugates), the form will be:
To be clear, here \(\vec{a}\) is the real part of the eigenvector, and \(\vec{b}\) is the imaginary part of the eigenvector.
For the above example (\(\lambda=\pm 2i\), eigenvector \(\vec{x}=[1,\pm i]\), this yields
Numerical solution to this example#
Remember, when we solve this numerically, we also need initial conditions. So let’s say that \(y_1(t=0)=2\) and \(y_2(t=0)=0.5\)
import numpy as np
from scipy.integrate import solve_ivp
import matplotlib.pyplot as plt
A = np.array([[0, 2],
[-2,0]])
def linear_homogeneous_diffeq(t, y):
return A@y
def linear_diffeq(t, y):
return [2*y[1],
-2*y[0]]
tspan = [0, 10]
y0 = [2, 0.5]
t_eval = np.linspace(0,10,100)
sol = solve_ivp(linear_homogeneous_diffeq, tspan, y0, t_eval=t_eval)
plt.plot(sol.t, sol.y.T,'o-')
plt.xlabel('Time t')
plt.ylabel('y')
Text(0, 0.5, 'y')
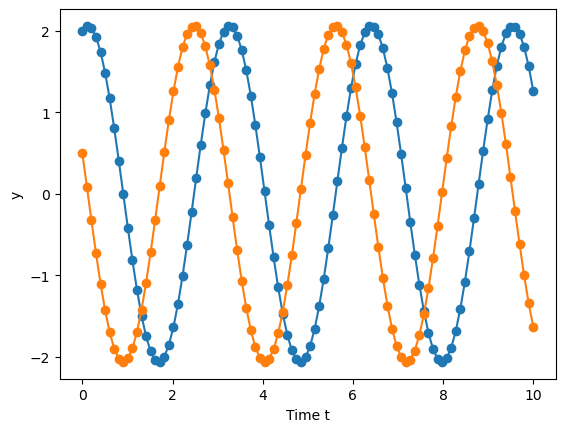
More than two coupled linear equations:#
Very easy to add more to our systems
\(\underline{\text{Ex}}\):
Note that equation 3 isn’t coupled, so in this case we can either solve that separately and plug into first two, or treat as 3D system:
The solution will be of the form:
where \(\lambda\)’s are the eigenvalues of \(\arr{A}\) and \(\vec{x}^{(i)}\)’s are the eigenvectors of \(\arr{A}\)
OR:
It’s easy to check the solution to \(y_3\) as it can be decoupled and is a solution to \(y_3' = y_3\).
Will need three initial conditions to find particular solution.
import numpy as np
# Quick numerical check to convince us that the eigenvalues/eigenvectors
# are correct above
A = np.array([[3,5,3],
[0,4,6],
[0,0,1]])
eigval,eigvec = np.linalg.eig(A)
print(eigval)
print(eigvec[:,2])
print(eigvec[:,2]*7/eigvec[0,2])
[3. 4. 1.]
[ 0.84270097 -0.48154341 0.24077171]
[ 7. -4. 2.]
Numerical solution#
We need three initial conditions now. Let’s say \(y_1(0)=4, y_2(0)=2, y_3(0)=6\). Almost no changes from the above code
import numpy as np
from scipy.integrate import solve_ivp
import matplotlib.pyplot as plt
A = np.array([[3, 5, 3],
[0, 4, 6],
[0, 0, 1]])
def linear_homogeneous_diffeq(t, y):
return A@y
tspan = [0, 1]
y0 = [4,2,6]
t_eval = np.linspace(0,1,100)
sol = solve_ivp(linear_homogeneous_diffeq, tspan, y0, t_eval=t_eval)
plt.plot(sol.t, sol.y.T,'o-')
plt.xlabel('Time t')
plt.ylabel('y')
Text(0, 0.5, 'y')
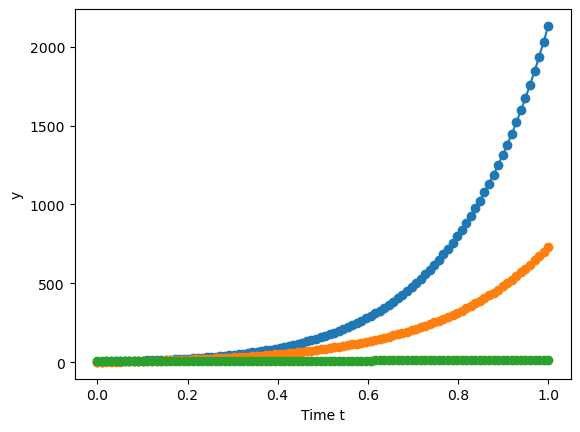
How do we handle non-homogeneous systems#
\(\rightarrow\) Same idea as single equations
Solve homogeneous system. From \(\arr{A}\):
Solve non-homogeneous part
Now, the particular solution \(\vec{y}_P\) is a vector
For this example use MoUC. Choose \(\vec{y}_P\) based on \(\vec{g} = \begin{bmatrix} 3\cos t \\ -2\cos t -3\sin t \end{bmatrix}\)
where \(\vec{u} = \begin{bmatrix} u_1 \\ u_2 \end{bmatrix}\) and \(\vec{v} = \begin{bmatrix} v_1 \\ v_2 \end{bmatrix}\) are the vectors of undetermined coefficients.
Then, \(\vec{y}_P' = \vec{u} \cos t - \vec{v}\sin t\)
Plug in expressions for \(\vec{y}_P\) and \(\vec{y}_P'\) into \(\vec{y}_P' = \arr{A}\vec{y}_P + \vec{g}\)
Match coefficients:
cos(t) terms (two equations):
* sin(t) terms (two equations):
\begin{align}
-\arr{A} \vec{u} -\vec{v} = \begin{bmatrix} 0 \\ -3 \end{bmatrix}
\end{align}
Solve system:
From first equation: \(\vec{u} = \begin{bmatrix} 3 \\ -2 \end{bmatrix} + \arr{A}\vec{v}\)
Plug into second equation:
and
Notice that this says the initial conditions don’t matter after a while!!
Numerical solutions#
import numpy as np
from scipy.integrate import solve_ivp
import matplotlib.pyplot as plt
A = np.array([[-3, 1],
[1,-3]])
def linear_nonhomogeneous_diffeq(t, y):
return A@y + [3*np.cos(t),
-2*np.cos(t)-3*np.sin(t)]
tspan = [0, 20]
y0 = [-100, 400000]
t_eval = np.linspace(0,20,100)
sol = solve_ivp(linear_nonhomogeneous_diffeq, tspan, y0, t_eval=t_eval)
plt.plot(sol.t, sol.y.T,'o-')
plt.xlabel('Time t')
plt.ylabel('y')
plt.ylim([-2,2])
(-2.0, 2.0)
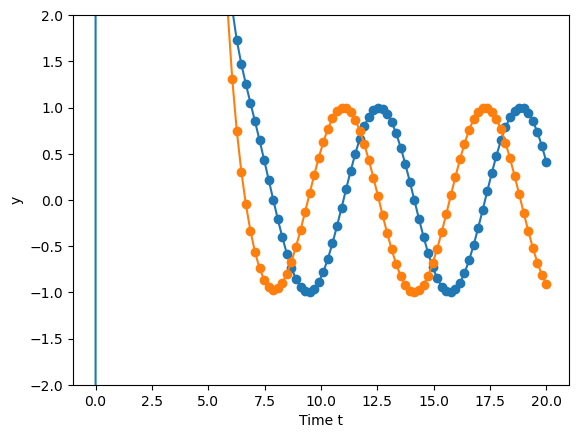
Non-homogeneous system - VOP Method#
Find the general solution to \(\vec{y}' = \begin{bmatrix}-2 & 1 \\ 1 & -2 \end{bmatrix}\vec{y} + \begin{bmatrix} 2e^{-t} \\ 3t \end{bmatrix}\)
same as:
Solve homogeneous system
from \(\arr{A} \rightarrow |\arr{A}-\lambda\arr{I}|=0\)
then \((\arr{A} - \lambda\arr{I})\vec{x} = \vec{0}\)
For \(\lambda_1=-3\)
For \(\lambda_2=-1\)
Then,
Solve non-homogeneous part using Variation of Parameters
Write the homogeneous solution as:
Just like with one dimensional case, we will form a particular solution by “replacing” the arbitrary constants of the homogeneous solution with an unknown function of \(t\), but this time with a vector. Assume \(\vec{y}_P(t) = \arr{Y}(t)\vec{u}(t)\)
We will determine \(\vec{u}(t)\) by substituting \(\vec{y}_P(t)\) into the original system
\(\rightarrow\) let’s derive in general terms
\(\implies\) because \(\arr{Y}\) is a solution to the homogeneous system, we know it satifies \(\arr{Y}' = \arr{A}\arr{Y}\rightarrow\) substitute this in
\(\rightarrow\) can multiply with inverse. We know \(\arr{Y}\) is non singular because \(\det(\arr{Y})=\) Wronskian which is non-zero for a basis
and
and
Now for our example:
we need \(\arr{Y}^{-1}(t)\). Can find using matrix property for a 2x2 matrix:
then
Then
Then,
Numerical solution#
Integrate \(\vec{y}' = \begin{bmatrix}-2 & 1 \\ 1 & -2 \end{bmatrix}\vec{y} + \begin{bmatrix} 2e^{-t} \\ 3t \end{bmatrix}\), with the initial condition \(\vec{y}(t=1)=[4,3]^T\) from t=1 to t=10.
import numpy as np
from scipy.integrate import solve_ivp
import matplotlib.pyplot as plt
A = np.array([[-2, 1],
[1,-2]])
def linear_nonhomogeneous_diffeq(t, y):
return A@y + [2*np.exp(-t),
3*t]
tspan = [1, 10]
y0 = [4, 3]
t_eval = np.linspace(1,10,100)
sol = solve_ivp(linear_nonhomogeneous_diffeq, tspan, y0, t_eval=t_eval)
plt.plot(sol.t, sol.y.T,'o-')
plt.xlabel('Time t')
plt.ylabel('y')
# plt.ylim([-2,2])
Text(0, 0.5, 'y')
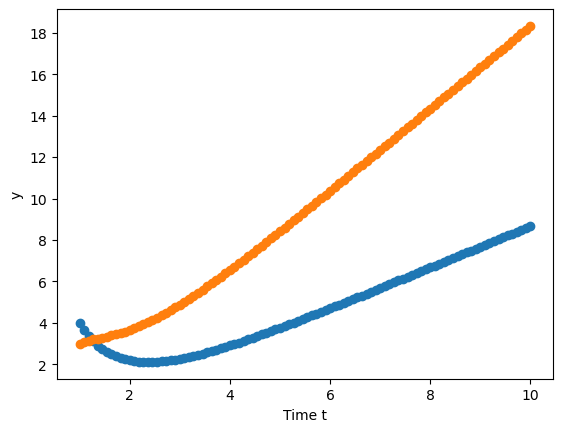