Boundary value problems
Contents
Boundary value problems#
Everything so far: Initial value problems
Initial conditions specify state at a single value of the independent variable
These IVP will have one unique solution. Implicitly, we have assumed that y and y’ are both defined at the initial condition, and that the system of differential equations is continuous over the solution interval.
\(\underline{\text{Ex}}\): \(y'' = 0 \hspace{2cm} y(0) = 2, \ y'(0) = 1\)
If we know information at two different points in the independent variable, we have a boundary value problem.
Example: pirate defense#
Analytical solution \(\rightarrow\) apply all of our boundary conditions
That third condition is very strange!
The conditions that we would use if we were treating this as an initial value problem are:
Say we are firing from a 100m embankment and the pirate ship is 200m away. We want to find initial conditions for \(v_x\) and \(v_z\) such that we hit the pirate ship. Code this up and let’s discuss. g = 9.8 [m/s^2] and r=0.1 [1/s]. Go ahead and try to solve this, starting from t=0 and integrating for 10 seconds.
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import solve_ivp
r = 0.1 #1/s
g = 9.8 #m/s^2
H = 100 # m
L = 200 # m
def diff_eq(t, y):
x, z, vx, vz = y
return [vx,
vz,
-r*vx,
-r*vz-g]
y0 = [0,
H,
52, #m/s
0] #m/s
t_span = [0,10]
t_eval = np.linspace(0,10,100)
sol = solve_ivp(diff_eq,
t_span=t_span,
y0=y0,
t_eval=t_eval)
plt.plot(sol.y[0,:],
sol.y[1,:])
plt.plot(200,0,'or')
plt.xlabel('x [m]')
plt.ylabel('z [m]')
Text(0, 0.5, 'z [m]')
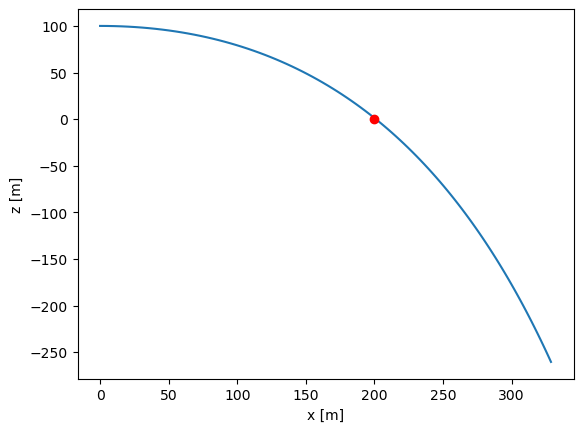
Say that we know that the cannonballs were fired at a speed of 40 m/s, but the angle of firing could be adjusted (e.g. \(v_x=v\cos \theta, v_z=v\sin\theta\)). Is it possible to hit the pirate ship?
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import solve_ivp
r = 0.1 #1/s
g = 9.8 #m/s^2
H = 100 # m
L = 200 # m
def diff_eq(t, y):
x, z, vx, vz = y
return [vx,
vz,
-r*vx,
-r*vz-g]
v0 = 40 # m/s
theta = 10 # degree
y0 = [0,
H,
v0*np.cos(theta/360*2*np.pi), #m/s
v0*np.sin(theta/360*2*np.pi)] #m/s
t_span = [0,10]
t_eval = np.linspace(0,10,100)
sol = solve_ivp(diff_eq,
t_span=t_span,
y0=y0,
t_eval=t_eval)
plt.plot(sol.y[0,:],
sol.y[1,:])
plt.plot(200,0,'or')
plt.xlabel('x [m]')
plt.ylabel('z [m]')
Text(0, 0.5, 'z [m]')
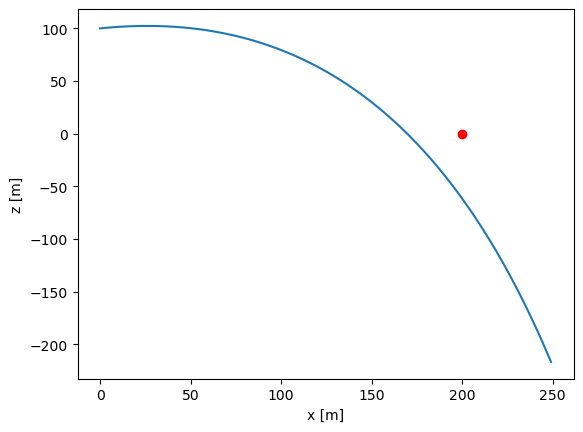
So, what did we learn?
Sometimes there is a solution for BVPs
Sometimes there are multiple solutions
Sometimes there are no solutions
There could even be infinite solutions
Analytical solutions to BVPs#
Analytical solutions to BVPs are usually not a problem to solve, just new boundary conditions.
\(\underline{\text{Ex}}\): \(2^{nd}\) order ODE would be specified by
Can have
no solutions
one solution
infinite solutions
\(\underline{\text{Ex}}\):
Example BVP:#
If we apply axial load or pressure, we will eventually have a buckle
As engineers, we care about shape
Also care about critical \(P\)
BC:
Two cases!
\(\lambda = 0\)
apply BC:
For \(\lambda = 0, \ y(x) = 0 \rightarrow\) trivial / no solution
This is the solution if \(P=0\)
2. \(\lambda \neq 0 \rightarrow y'''' + \lambda y'' = 0\)
guess \(y(x) = e^{rx}\)
plug in:
BC:
1.\begin{align} y(x=0) = 0 = c_1 + c_4 \end{align} 2.\begin{align} y’’(x=0) = 0 = -c_4 \lambda \rightarrow c_4 = 0, \ c_1 = 0 \end{align} 3.\begin{align} y(x=L) = 0 &= c_2L + c_3\sin\sqrt{\lambda}L\ c_2 & = -\frac{1}{L}c_3\sin\sqrt{\lambda}L \end{align} 4.\begin{align} y’(x=L) = 0 &= c_2 + c_3\sqrt{\lambda}\cos\sqrt{\lambda}L\ 0 &= -\frac{1}{L}c_3\sin\sqrt{\lambda}L + c_3\sqrt{\lambda}\cos\sqrt{\lambda}L\ &= c_3(\sqrt{\lambda}L\cos\sqrt{\lambda}L - \sin\sqrt{\lambda}L) \end{align} Only situation without trivial solution is if
\(\rightarrow\) infinite number of solutions but only care about the first one (smallest \(P\))
from scipy.optimize import root
import numpy as np
import matplotlib.pyplot as plt
L = 1
sol = root(lambda x: np.tan(x)-x, 4+np.pi*4)
rootLambdaL = sol.x
lambdaSol = (rootLambdaL/L)**2
c3 = 2
c2 = -c3*np.sin(np.sqrt(lambdaSol)*L)/L
xrange = np.linspace(0,L,100)
plt.plot(xrange,
c2*xrange+c3*np.sin(np.sqrt(lambdaSol)*xrange))
plt.plot(0,0,'ok')
plt.plot([0.9,1],[0,0],'-k')
plt.xlabel('x')
plt.ylabel('y')
Text(0, 0.5, 'y')
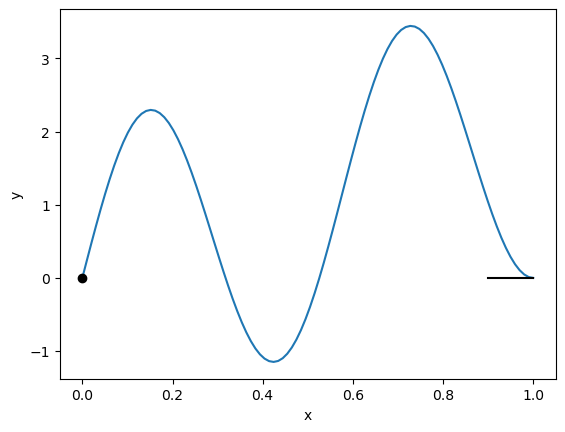
plt.xlabel('x')
Text(0.5, 0, 'x')
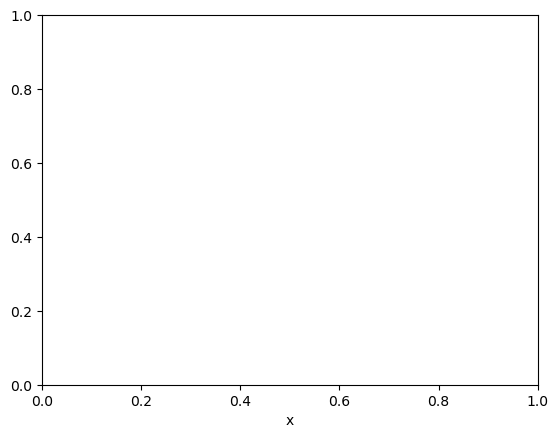